Google Sign In iOS 1.0.0
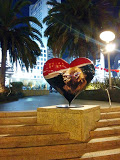
The new Google Sign In SDK for iOS is out! With a new version number, and separated from the old Google+ SDK, the Sign-In SDK should make it easier and faster to implement Google Sign-In in your app. Lets take a look at how to use it from Swift.Unfortunately the library isn't available from Cocoapods yet, so you'll have to drop it in manually. Setup is pretty easy: add in the GoogleSignIn.framework from the zip download and add the system AddressBook and SystemConfiguration frameworks. If you want to use the supplied button, you'll also need to add the GoogleSignIn.bundle from the SDK zip which contains the fonts, images and translations for the standard button - using the Add Files to "project" menu option should automatically set it in the Copy Bundle Resources part of your build step.If your sign in button is invisible when you launch the app, you probably haven't copied the GoogleSignIn.bundle from the SDK zip file.In the Build Settings phase, add -ObjC i…